Java String contains()
method returns true
if this string contains the given character sequence.
Table of Contents
Java String contains() method signature
String contains() method signature is:
public boolean contains(CharSequence s)
There are three classes that implements CharSequence interface.
- String
- StringBuffer
- StringBuilder
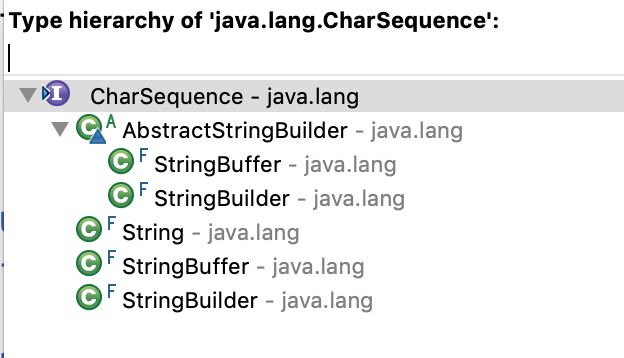
Important Points for contains() method
- This utility method was introduced in Java 1.5
- It uses
indexOf()
method to check if this string contains the argument string or not. - If the argument is
null
thenNullPointerException
will be thrown. - This method is case sensitive. For example,
"x".contains("X");
will returnfalse
. - You can convert both the strings to lowercase for case insensitive operation. For example,
"x".toLowerCase().contains("X".toLowerCase());
will return true.
String contains() Examples
- Let’s look at a simple example where we pass String as an argument.
- We can create string objects using Unicode values. The unicode value of ‘H’ is ‘\u0048’.
- Let’s look at an example with StringBuffer object as the argument.
- Let’s look at an example with StringBuilder object as the argument.
- The
java.nio.CharBuffer
class implements CharSequence interface. Let’s look at an example of contains() method with CharBuffer object as argument.
String s = "Hello World";
System.out.println(s.contains("Hello")); // true
System.out.println(s.contains("\u0048")); // true
System.out.println(s.contains(new StringBuffer("Hello"))); // true
System.out.println(s.contains(new StringBuilder("Hello"))); // true
CharBuffer cb = CharBuffer.allocate(5);
cb.append('H');cb.append('e');cb.append('l');cb.append('l');cb.append('o');
cb.clear();
System.out.println(cb); // Hello
System.out.println(s.contains(cb)); // true
JShell Examples of contains() method
We can run above code snippets in JShell too.
jshell> String s = "Hello World";
s ==> "Hello World"
jshell> s.contains("Hello");
$20 ==> true
jshell> s.contains("\u0048");
$21 ==> true
jshell> s.contains(new StringBuffer("Hello"));
$22 ==> true
jshell> s.contains(new StringBuilder("Hello"));
$23 ==> true
jshell> import java.nio.CharBuffer;
...>
jshell> CharBuffer cb = CharBuffer.allocate(5);
cb ==>
jshell> cb.append('H');cb.append('e');cb.append('l');cb.append('l');cb.append('o');
$26 ==>
$27 ==>
$28 ==>
$29 ==>
$30 ==>
jshell> cb.clear();
$31 ==> Hello
jshell> System.out.println(cb);
Hello
jshell> s.contains(cb)
$33 ==> true
jshell>